- Download java via http://www.java.com/en/download/
- Install
- Setting up the path
>>>Command prompt to setting up the path<<<
- First go to java installed folder --> jdkx.x.x_xx -->bin and copy the path
- Then open command prompt ([Window key+R] then type cmd)
- Go to the folder you expected to do your java work using cmd.
(*See the note below) - Then type, set path=%path%;your bin path copied
eg. set path=%path%;C:\Program Files\Java\jdk1.5.0_09\bin
- to change the drive, type the drive name: eg. D:
- to change the folder type cd folderName eg. cd Umesh
- to clear the path and go back to the drive type cd/
OR
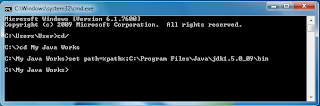
>>>>>>Advaced Settings<<<<<<
Windows 8
- Drag the Mouse pointer to the Right bottom corner of the screen
- Click on the Search icon and type: Control Panel
- Click on -> Control Panel -> System -> Advanced
- Click on Environment Variables, under System Variables, find PATH, and click on it.
- In the Edit windows, modify PATH by adding the location of the class to the value for PATH. If you do not have the item PATH, you may select to add a new variable and add PATH as the name and the location of the class as the value.
- Close the window.
- Reopen Command prompt window, and run your java code.
Windows 7
- Select Computer from the Start menu
- Choose System Properties from the context menu
- Click Advanced system settings > Advanced tab
- Click on Environment Variables, under System Variables, find PATH, and click on it.
- In the Edit windows, modify PATH by adding the location of the class to the value for PATH. If you do not have the item PATH, you may select to add a new variable and add PATH as the name and the location of the class as the value.
- Reopen Command prompt window, and run your java code.
Windows XP
- Start -> Control Panel -> System -> Advanced
- Click on Environment Variables, under System Variables, find PATH, and click on it.
- In the Edit windows, modify PATH by adding the location of the class to the value for PATH. If you do not have the item PATH, you may select to add a new variable and add PATH as the name and the location of the class as the value.
- Close the window.
- Reopen Command prompt window, and run your java code.
Windows Vista
- Right click My Computer icon
- Choose Properties from the context menu
- Click Advanced tab (Advanced system settings link in Vista)
- In the Edit windows, modify PATH by adding the location of the class to the value for PATH. If you do not have the item PATH, you may select to add a new variable and add PATH as the name and the location of the class as the value.
- Reopen Command prompt window, and run your java code.
Setting Path on Solaris and Linux
To find out if the java executable is in your PATH, execute:% java -version
This will print the version of the java executable, if it can find it. If you get error java: Command not found. Then path is not properly set.
To find out which java executable the first one found in your PATH, execute:
% which java
Below are the steps to set the PATH permanently,
Note: We are here giving instructions for two most popular Shells on Linux and Solaris.
Please visit link below if you are using any other shells.
Path Setting Tutorial
For bash Shell:
- Edit the startup file (~/ .bashrc)
- Modify PATH variable:
PATH="$PATH":/usr/local/jdk1.6.0/bin
- export PATH
- Save and close the file
- Open new Terminal window
- Verify the PATH is set properly
% java -version
For C Shell (csh):
- Edit startup file (~/ .cshrc)
- Set Path
set path="$PATH":/usr/local/jdk1.6.0/bin
- Save and Close the file
- Open new Terminal window
- Verify the PATH is set properly
% java -version